Zustand Makes React Too Easy
React has revolutionized the way we build user interfaces, but managing state can still be a challenge. Traditional state management solutions like Redux can be complex and verbose. Enter Zustand, a small, fast, and scalable state management library that makes managing state in React applications a breeze. In this article, we'll explore how Zustand simplifies state management and why it's becoming a popular choice among developers. We'll also provide examples using TypeScript to demonstrate its power and flexibility. Introduction to Zustand Zustand is a minimalistic state management library for React that focuses on simplicity and performance. It provides a straightforward API for creating and managing state, making it easy to integrate into any React application. Unlike Redux, Zustand does not require boilerplate code or complex setup, making it an ideal choice for small to medium-sized applications. Key Features of Zustand Simple API: Zustand offers a simple and intuitive API for creating and managing state. TypeScript Support: Zustand has built-in TypeScript support, making it easy to use in TypeScript projects. Performance: Zustand is designed to be fast and efficient, with minimal overhead. Flexibility: Zustand can be used with any React application, regardless of its size or complexity. Getting Started with Zustand To get started with Zustand, you need to install the library using npm or yarn: npm install zustand or yarn add zustand Creating a Store with Zustand Creating a store with Zustand is straightforward. You define a store using the create function and specify the initial state and any actions you want to perform on that state. Example: Basic Counter Store Let's create a simple counter store using Zustand and TypeScript. import create from 'zustand'; interface CounterState { count: number; increment: () => void; decrement: () => void; } const useCounterStore = create((set) => ({ count: 0, increment: () => set((state) => ({ count: state.count + 1 })), decrement: () => set((state) => ({ count: state.count - 1 })), })); export default useCounterStore; In this example, we define a CounterState interface to specify the shape of our state and the actions we want to perform. We then use the create function to create the store, passing in a function that returns the initial state and the actions. Using the Store in a Component Now that we have our store, we can use it in a React component. Zustand provides a hook called useStore that allows you to access the state and actions from the store. import React from 'react'; import useCounterStore from './useCounterStore'; const Counter: React.FC = () => { const { count, increment, decrement } = useCounterStore(); return ( Count: {count} Increment Decrement ); }; export default Counter; In this example, we use the useCounterStore hook to access the count, increment, and decrement properties from the store. We then use these properties to display the current count and provide buttons to increment and decrement the count. Advanced State Management with Zustand Zustand is not just for simple state management. It can also handle more complex scenarios, such as nested state, derived state, and asynchronous actions. Example: Todo List with Nested State Let's create a more complex example: a todo list with nested state. import create from 'zustand'; interface Todo { id: number; text: string; completed: boolean; } interface TodoState { todos: Todo[]; addTodo: (text: string) => void; toggleTodo: (id: number) => void; removeTodo: (id: number) => void; } const useTodoStore = create((set) => ({ todos: [], addTodo: (text) => set((state) => ({ todos: [...state.todos, { id: Date.now(), text, completed: false }], })), toggleTodo: (id) => set((state) => ({ todos: state.todos.map((todo) => todo.id === id ? { ...todo, completed: !todo.completed } : todo ), })), removeTodo: (id) => set((state) => ({ todos: state.todos.filter((todo) => todo.id !== id), })), })); export default useTodoStore; In this example, we define a Todo interface to specify the shape of a todo item and a TodoState interface to specify the shape of our state and the actions we want to perform. We then use the create function to create the store, passing in a function that returns the initial state and the actions. Using the Todo Store in a Component Now that we have our todo store, we can use it in a React component. import React, { useState } from 'react'; import useTodoStore from './useTodoStore'; const TodoList: React.FC = () => { const { todos, addTodo, toggleTodo, removeTodo } = useTodoStore(); const [newTodo, setNewTodo] = useState(''); const handleAddTodo = () => { if (newTodo.trim()) { addTodo(newTodo); setNewTodo(''

React has revolutionized the way we build user interfaces, but managing state can still be a challenge. Traditional state management solutions like Redux can be complex and verbose. Enter Zustand, a small, fast, and scalable state management library that makes managing state in React applications a breeze. In this article, we'll explore how Zustand simplifies state management and why it's becoming a popular choice among developers. We'll also provide examples using TypeScript to demonstrate its power and flexibility.
Introduction to Zustand
Zustand is a minimalistic state management library for React that focuses on simplicity and performance. It provides a straightforward API for creating and managing state, making it easy to integrate into any React application. Unlike Redux, Zustand does not require boilerplate code or complex setup, making it an ideal choice for small to medium-sized applications.
Key Features of Zustand
- Simple API: Zustand offers a simple and intuitive API for creating and managing state.
- TypeScript Support: Zustand has built-in TypeScript support, making it easy to use in TypeScript projects.
- Performance: Zustand is designed to be fast and efficient, with minimal overhead.
- Flexibility: Zustand can be used with any React application, regardless of its size or complexity.
Getting Started with Zustand
To get started with Zustand, you need to install the library using npm or yarn:
npm install zustand
or
yarn add zustand
Creating a Store with Zustand
Creating a store with Zustand is straightforward. You define a store using the create
function and specify the initial state and any actions you want to perform on that state.
Example: Basic Counter Store
Let's create a simple counter store using Zustand and TypeScript.
import create from 'zustand';
interface CounterState {
count: number;
increment: () => void;
decrement: () => void;
}
const useCounterStore = create<CounterState>((set) => ({
count: 0,
increment: () => set((state) => ({ count: state.count + 1 })),
decrement: () => set((state) => ({ count: state.count - 1 })),
}));
export default useCounterStore;
In this example, we define a CounterState
interface to specify the shape of our state and the actions we want to perform. We then use the create
function to create the store, passing in a function that returns the initial state and the actions.
Using the Store in a Component
Now that we have our store, we can use it in a React component. Zustand provides a hook called useStore
that allows you to access the state and actions from the store.
import React from 'react';
import useCounterStore from './useCounterStore';
const Counter: React.FC = () => {
const { count, increment, decrement } = useCounterStore();
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
};
export default Counter;
In this example, we use the useCounterStore
hook to access the count
, increment
, and decrement
properties from the store. We then use these properties to display the current count and provide buttons to increment and decrement the count.
Advanced State Management with Zustand
Zustand is not just for simple state management. It can also handle more complex scenarios, such as nested state, derived state, and asynchronous actions.
Example: Todo List with Nested State
Let's create a more complex example: a todo list with nested state.
import create from 'zustand';
interface Todo {
id: number;
text: string;
completed: boolean;
}
interface TodoState {
todos: Todo[];
addTodo: (text: string) => void;
toggleTodo: (id: number) => void;
removeTodo: (id: number) => void;
}
const useTodoStore = create<TodoState>((set) => ({
todos: [],
addTodo: (text) => set((state) => ({
todos: [...state.todos, { id: Date.now(), text, completed: false }],
})),
toggleTodo: (id) => set((state) => ({
todos: state.todos.map((todo) =>
todo.id === id ? { ...todo, completed: !todo.completed } : todo
),
})),
removeTodo: (id) => set((state) => ({
todos: state.todos.filter((todo) => todo.id !== id),
})),
}));
export default useTodoStore;
In this example, we define a Todo
interface to specify the shape of a todo item and a TodoState
interface to specify the shape of our state and the actions we want to perform. We then use the create
function to create the store, passing in a function that returns the initial state and the actions.
Using the Todo Store in a Component
Now that we have our todo store, we can use it in a React component.
import React, { useState } from 'react';
import useTodoStore from './useTodoStore';
const TodoList: React.FC = () => {
const { todos, addTodo, toggleTodo, removeTodo } = useTodoStore();
const [newTodo, setNewTodo] = useState('');
const handleAddTodo = () => {
if (newTodo.trim()) {
addTodo(newTodo);
setNewTodo('');
}
};
return (
<div>
<input
type="text"
value={newTodo}
onChange={(e) => setNewTodo(e.target.value)}
placeholder="Add a new todo"
/>
<button onClick={handleAddTodo}>Add Todo</button>
<ul>
{todos.map((todo) => (
<li key={todo.id}>
<input
type="checkbox"
checked={todo.completed}
onChange={() => toggleTodo(todo.id)}
/>
{todo.text}
<button onClick={() => removeTodo(todo.id)}>Remove</button>
</li>
))}
</ul>
</div>
);
};
export default TodoList;
In this example, we use the useTodoStore
hook to access the todos
, addTodo
, toggleTodo
, and removeTodo
properties from the store. We then use these properties to display the list of todos and provide inputs and buttons to add, toggle, and remove todos.
Asynchronous Actions with Zustand
Zustand also supports asynchronous actions, making it easy to handle data fetching and other asynchronous operations.
Example: Fetching Data from an API
Let's create an example where we fetch data from an API and store it in our Zustand store.
import create from 'zustand';
interface DataState {
data: any[];
loading: boolean;
error: string | null;
fetchData: () => Promise<void>;
}
const useDataStore = create<DataState>((set) => ({
data: [],
loading: false,
error: null,
fetchData: async () => {
set({ loading: true, error: null });
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
set({ data, loading: false });
} catch (error) {
set({ error: error.message, loading: false });
}
},
}));
export default useDataStore;
In this example, we define a DataState
interface to specify the shape of our state and the actions we want to perform. We then use the create
function to create the store, passing in a function that returns the initial state and the fetchData
action.
Using the Data Store in a Component
Now that we have our data store, we can use it in a React component.
import React, { useEffect } from 'react';
import useDataStore from './useDataStore';
const DataFetcher: React.FC = () => {
const { data, loading, error, fetchData } = useDataStore();
useEffect(() => {
fetchData();
}, [fetchData]);
if (loading) {
return <div>Loading...</div>;
}
if (error) {
return <div>Error: {error}</div>;
}
return (
<div>
<ul>
{data.map((item: any) => (
<li key={item.id}>{item.name}</li>
))}
</ul>
</div>
);
};
export default DataFetcher;
In this example, we use the useDataStore
hook to access the data
, loading
, error
, and fetchData
properties from the store. We then use these properties to display the list of data items and handle loading and error states.
Conclusion
Zustand is a powerful and flexible state management library that makes managing state in React applications easy and efficient. With its simple API, built-in TypeScript support, and performance optimizations, Zustand is an excellent choice for small to medium-sized applications. Whether you're building a simple counter, a complex todo list, or fetching data from an API, Zustand has you covered.
By leveraging Zustand, you can simplify your state management, reduce boilerplate code, and focus on building great user experiences. Give Zustand a try in your next React project and see how it can make your development process smoother and more enjoyable.
Happy coding!
What's Your Reaction?
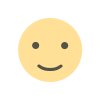
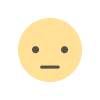
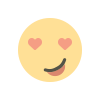
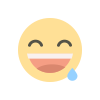
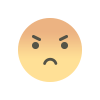
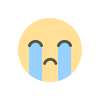
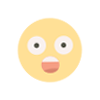