Why doesn't dimensionResource in Jetpack Compose use remember, and what happens if we add it?
In Jetpack Compose, we use **dimensionResource **to retrieve a dimension value from resources and convert it to Dp. The built-in function is defined as: @Composable @ReadOnlyComposable fun dimensionResource(@DimenRes id: Int): Dp { val context = LocalContext.current val density = LocalDensity.current val pxValue = context.resources.getDimension(id) return Dp(pxValue / density.density) } This function does not use remember. My questions: Why doesn't dimensionResource internally use remember to cache the result and prevent redundant recomputation during recomposition? What would happen if I wrapped it in remember like this? @Composable fun rememberedDimensionResource(@DimenRes id: Int): Dp { val context = LocalContext.current val density = LocalDensity.current return remember(id) { val pxValue = context.resources.getDimension(id) Dp(pxValue / density.density) } } Would this improve performance by avoiding unnecessary resource lookups, or is there a reason why dimensionResource is designed without remember? I'm trying to understand the implications of caching dimension values in Jetpack Compose and whether this would provide a real optimization. Any insights would be greatly appreciated.
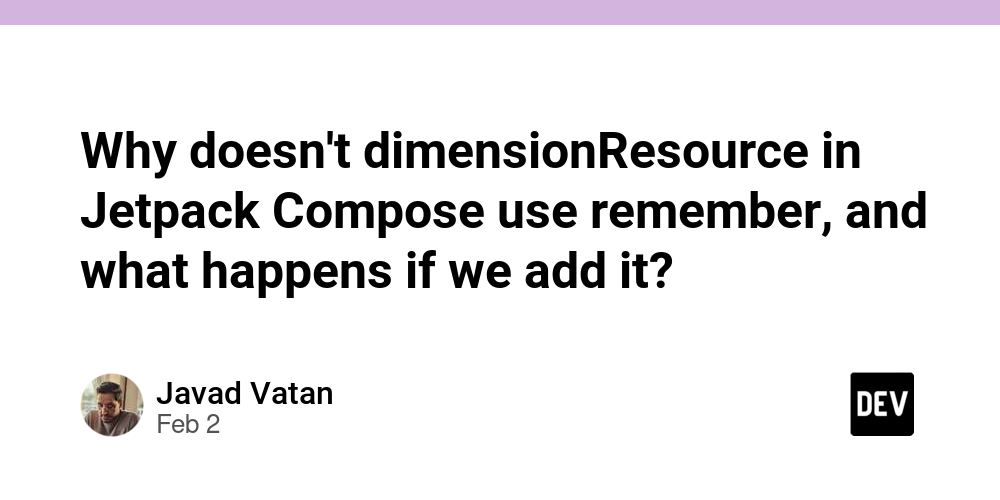
In Jetpack Compose, we use **dimensionResource **to retrieve a dimension value from resources and convert it to Dp. The built-in function is defined as:
@Composable
@ReadOnlyComposable
fun dimensionResource(@DimenRes id: Int): Dp {
val context = LocalContext.current
val density = LocalDensity.current
val pxValue = context.resources.getDimension(id)
return Dp(pxValue / density.density)
}
This function does not use remember.
My questions:
Why doesn't dimensionResource internally use remember to cache the
result and prevent redundant recomputation during recomposition?What would happen if I wrapped it in remember like this?
@Composable
fun rememberedDimensionResource(@DimenRes id: Int): Dp {
val context = LocalContext.current
val density = LocalDensity.current
return remember(id) {
val pxValue = context.resources.getDimension(id)
Dp(pxValue / density.density)
}
}
Would this improve performance by avoiding unnecessary resource lookups, or is there a reason why dimensionResource is designed without remember?
I'm trying to understand the implications of caching dimension values in Jetpack Compose and whether this would provide a real optimization.
Any insights would be greatly appreciated.