How to Fetch and Display Blog Articles from Contentful in a Next.js Application
In my previous blog post, I explained how to connect your Next.js application to Contentful CMS, where you can store and manage your blog articles. In this post, I’ll show you how to fetch those articles, retrieve specific data like the title and publication date, and display them on your Next.js app using server-side rendering (SSR). Overview Before diving into the details, let me quickly summarize what you’ve done so far and explain the workflow. What You’ve Accomplished In the previous blog post, you: Set up Contentful CMS to host your blog articles. Created a Content Type with fields like title and description to store article-specific data. Built a Next.js application that serves as the front end to display these articles to your audience. Now, it’s time to fetch the articles you’ve added to your CMS and display them on your application’s page, making them accessible to readers. The Goal For this example, let’s create a page at /pages/articles/index.js where you’ll display a list of articles with the titleand publication date. How to Display Articles Your Contentful Content Type automatically provides an endpoint that contains your articles. To display these articles in your application, you need to fetch the data from this endpoint and render it on your page. There are two main ways to fetch data in a Next.js application: Client-Side Rendering (CSR) Server-Side Rendering (SSR) Why SSR is the Better Choice If you fetch data using Client-Side Rendering (CSR), all the data will be loaded directly into the client’s browser. This includes every single article stored in your Contentful CMS, not just the one currently being viewed. For example, if a user is reading an article titled "How to Create a Next.js App", their browser will load not only this article but all other articles as well. This approach has several drawbacks: Security Concerns: The endpoint where your articles are stored will be exposed in the browser, making it accessible to anyone. Poor SEO: Search engines cannot effectively crawl your content if it is fetched on the client side (More about SEO read: The Ultimate Guide to SEO Optimization in Next.js for Beginners). To demonstrate this, you can inspect the network requests in your browser (e.g., Chrome > Inspect > Network). You’ll notice that every article is downloaded to the client’s browser, even though the user only sees one article on the page. For these reasons, Server-Side Rendering (SSR) is the recommended approach. With SSR, the data fetching happens on the server, and only the requested data is sent to the client. Fetching Articles with SSR Below is an example of how to fetch and display articles using SSR in a Next.js application. The following code will render the titles and publication dates of your blog articles on the /pages/articles/index.js page: // Import the Contentful client import { client } from "@/lib/contentful"; // Fetch data from Contentful during SSR export async function getServerSideProps() { const response = await client.getEntries({ content_type: 'myblogs', // Replace with your Content Model ID select: 'fields.title,fields.date', // Only fetch title and date fields }); const articles = response.items.map((item) => { const rawDate = new Date(item.fields.date); const formattedDate = `${rawDate.getDate().toString().padStart(2, '0')}/${(rawDate.getMonth() + 1) .toString() .padStart(2, '0')}/${rawDate.getFullYear()}`; return { title: item.fields.title, date: formattedDate, }; }); return { props: { articles }, }; } // Render the articles on the page export default function ArticlesPage({ articles }) { return ( Blog Articles {articles.map((article, index) => ( {article.title} Published Date: {article.date} ))} ); } Explanation of the Code Fetching Data on the Server The getServerSideProps function is used to fetch articles from Contentful during server-side rendering. Only the title and date fields are fetched to minimize the amount of data sent to the client. 2.Formatting Dates The raw date retrieved from Contentful is formatted into the DD/MM/YYYY format on the server side. This ensures that the client receives properly formatted dates, preventing any hydration errors or mismatched content between the server and client. 3.Displaying Data The ArticlesPage component renders the fetched articles as a list. Each list item includes the article’s title and publication date Next Steps Once your articles are displayed on the /pages/articles/index.js page, the next step is to create dynamic pages for individual articles. For example, clicking on an article’s title could take the user to /pages/articles/how-to-create-nextjs-app, where they can read the full article. To achieve this, you’ll need to set up dynamic routes in Next.js and use t

In my previous blog post, I explained how to connect your Next.js application to Contentful CMS, where you can store and manage your blog articles.
In this post, I’ll show you how to fetch those articles, retrieve specific data like the title and publication date, and display them on your Next.js app using server-side rendering
(SSR).
Overview
Before diving into the details, let me quickly summarize what you’ve done so far and explain the workflow.
What You’ve Accomplished
In the previous blog post, you:
Set up Contentful CMS to host your blog articles.
Created a Content Type with fields like title and description to store article-specific data.
Built a Next.js application that serves as the front end to display these articles to your audience.
Now, it’s time to fetch the articles you’ve added to your CMS
and display them on your application’s page, making them accessible to readers.
The Goal
For this example, let’s create a page at /pages/articles/index.js
where you’ll display a list of articles with the titleand publication date.
How to Display Articles
Your Contentful Content Type automatically provides an endpoint that contains your articles. To display these articles in your application, you need to fetch the data from this endpoint and render it on your page.
There are two main ways to fetch data in a Next.js
application:
- Client-Side Rendering (CSR)
- Server-Side Rendering (SSR)
Why SSR is the Better Choice
If you fetch data using Client-Side Rendering
(CSR), all the data will be loaded directly into the client’s browser. This includes every single article stored in your Contentful CMS, not just the one currently being viewed.
For example, if a user is reading an article titled "How to Create a Next.js App", their browser will load not only this article but all other articles as well. This approach has several drawbacks:
Security Concerns: The endpoint where your articles are stored will be exposed in the browser, making it accessible to anyone.
Poor SEO: Search engines cannot effectively crawl your content if it is fetched on the client side (More about SEO read: The Ultimate Guide to SEO Optimization in Next.js for Beginners).
To demonstrate this, you can inspect the network requests in your browser (e.g., Chrome > Inspect > Network). You’ll notice that every article is downloaded to the client’s browser, even though the user only sees one article on the page.
For these reasons, Server-Side Rendering (SSR) is the recommended approach. With SSR, the data fetching happens on the server, and only the requested data is sent to the client.
Fetching Articles with SSR
Below is an example of how to fetch and display articles using SSR in a Next.js application. The following code will render the titles and publication dates of your blog articles on the /pages/articles/index.js page:
// Import the Contentful client
import { client } from "@/lib/contentful";
// Fetch data from Contentful during SSR
export async function getServerSideProps() {
const response = await client.getEntries({
content_type: 'myblogs', // Replace with your Content Model ID
select: 'fields.title,fields.date', // Only fetch title and date fields
});
const articles = response.items.map((item) => {
const rawDate = new Date(item.fields.date);
const formattedDate = `${rawDate.getDate().toString().padStart(2, '0')}/${(rawDate.getMonth() + 1)
.toString()
.padStart(2, '0')}/${rawDate.getFullYear()}`;
return {
title: item.fields.title,
date: formattedDate,
};
});
return {
props: { articles },
};
}
// Render the articles on the page
export default function ArticlesPage({ articles }) {
return (
Blog Articles
{articles.map((article, index) => (
-
{article.title}
Published Date: {article.date}
))}
);
}
Explanation of the Code
- Fetching Data on the Server The getServerSideProps function is used to fetch articles from Contentful during server-side rendering. Only the title and date fields are fetched to minimize the amount of data sent to the client.
2.Formatting Dates
The raw date retrieved from Contentful is formatted into the DD/MM/YYYY
format on the server side. This ensures that the client receives properly formatted dates, preventing any hydration errors or mismatched content between the server and client.
3.Displaying Data
The ArticlesPage component renders the fetched articles as a list. Each list item includes the article’s title
and publication date
Next Steps
Once your articles are displayed on the /pages/articles/index.js
page, the next step is to create dynamic pages for individual articles.
For example, clicking on an article’s title could take the user to /pages/articles/how-to-create-nextjs-app
, where they can read the full article. To achieve this, you’ll need to set up dynamic routes in Next.js and use the slug field from your Contentful Content Type.
I’ll cover how to create dynamic routes and generate individual article pages in my next blog post.
If you found this guide helpful, be sure to follow my blog for more content.
What's Your Reaction?
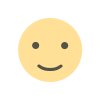
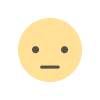
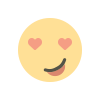
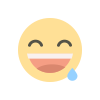
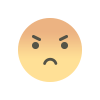
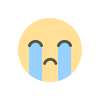
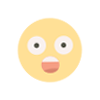